Best Strategies for Writing Modular Code in Any Language
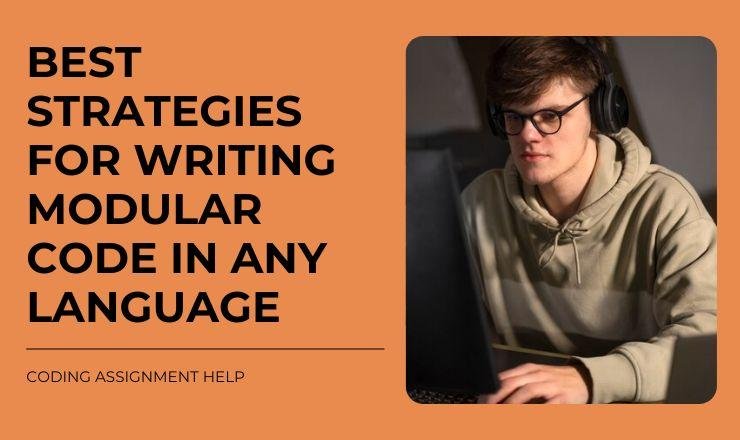
In the realm of software development, writing modular code is a crucial practice that enhances maintainability, readability, and reusability. Whether you are working on small projects or large-scale enterprise applications, modular programming ensures efficiency and ease of debugging. This article will explore the best strategies for writing modular code in any programming language while integrating essential keywords like coding assignment help, Programming Assignment Help, and online programming assignment help.
Understanding Modular Code
Modular programming is an approach where a complex program is divided into smaller, independent, and reusable modules. Each module performs a specific task and can be integrated with other modules to build a complete application. This methodology enhances scalability, simplifies debugging, and allows for code reuse across multiple projects.
Benefits of Writing Modular Code
-
Reusability: Redundancy can be decreased by reusing modules in many programs.
-
Maintainability: Debugging and modifying specific modules is easier than working with monolithic code.
-
Scalability: Applications can grow efficiently by adding new modules without affecting existing ones.
-
Collaboration: Different developers can work on separate modules simultaneously.
-
Readability: Well-structured modular code is easier to understand and document.
For students and professionals seeking coding assignment help, understanding these advantages is essential to producing clean and efficient code.
Strategies for Writing Modular Code
1. Follow the Single Responsibility Principle (SRP)
Every module should have a single responsibility. If a module performs multiple tasks, it becomes complex and harder to maintain. Breaking down tasks into individual functions ensures better modularity.
Example in Python:
# Bad Practice: Single function performing multiple tasks
class UserManager:
def handle_user(self, user):
self.validate_user(user)
self.save_to_db(user)
self.send_email(user)
# Good Practice: Separate functions for different tasks
class UserManager:
def validate_user(self, user):
# Validation logic
pass
def save_to_db(self, user):
# Database logic
pass
def send_email(self, user):
# Email sending logic
pass
Students looking for programming assignment help in Australia should ensure that their code adheres to SRP for better organization and maintainability.
2. Use Functions and Methods Effectively
Breaking down large blocks of code into smaller functions improves readability and maintainability. Functions should perform a single task and have descriptive names.
Example in Java:
// Inefficient Code
public void processUser(User user) {
if (user.getAge() > 18) {
System.out.println("User is eligible");
} else {
System.out.println("User is not eligible");
}
}
// Modular Code
public boolean isUserEligible(User user) {
return user.getAge() > 18;
}
public void displayEligibility(User user) {
if (isUserEligible(user)) {
System.out.println("User is eligible");
} else {
System.out.println("User is not eligible");
}
}
3. Encapsulation and Data Hiding
Encapsulation ensures that data is protected and only accessible through well-defined interfaces. This prevents unintended modifications and enhances security.
Example in C++:
class Account {
private:
double balance;
public:
Account(double initialBalance) { balance = initialBalance; }
double getBalance() { return balance; }
void deposit(double amount) { balance += amount; }
};
4. Use Object-Oriented Programming (OOP) Principles
Object-Oriented Programming helps in structuring code into objects that interact with each other, improving modularity and code reuse.
Key OOP concepts:
-
Encapsulation: Hiding internal state
-
Inheritance: Code reuse through parent-child relationships
-
Polymorphism: Using common interfaces for different implementations
5. Adopt a Modular File Structure
Organizing files into relevant directories improves project manageability. For example, in a Python Flask application:
/project-root
/app
/models
/controllers
/views
/static
/templates
/config.py
/run.py
This structure ensures clear separation of concerns, benefiting those seeking online programming assignment help.
6. Use Dependency Injection
Dependency injection allows modules to be loosely coupled, making code easier to test and modify.
Example in Java:
public class EmailService {
public void sendEmail(String message) {
System.out.println("Sending email: " + message);
}
}
public class UserService {
private EmailService emailService;
public UserService(EmailService emailService) {
this.emailService = emailService;
}
public void notifyUser() {
emailService.sendEmail("Notification Message");
}
}
7. Write Unit Tests for Each Module
Unit testing ensures that individual modules function correctly before integrating them into the main application. Frameworks like JUnit (Java), PyTest (Python), and Mocha (JavaScript) are helpful.
Example in Python:
import unittest
from mymodule import add_numbers
class TestMathFunctions(unittest.TestCase):
def test_add_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
if __name__ == '__main__':
unittest.main()
8. Leverage Version Control Systems
Using Git for version control ensures that modular code is tracked, allowing developers to revert changes if necessary. Best practices include:
-
Using meaningful commit messages
-
Creating feature branches for different modules
-
Conducting code reviews before merging
9. Refactor Code Regularly
Refactoring helps improve code structure without changing its functionality. Regularly reviewing and improving code enhances efficiency and performance.
10. Use Design Patterns
Design patterns like Singleton, Factory, and Observer help create scalable and modular applications. Learning these patterns is valuable for those seeking programming assignment help in Australia.
Conclusion
Writing modular code is essential for creating maintainable, scalable, and efficient applications. By following principles like SRP, encapsulation, dependency injection, and proper file structuring, developers can significantly improve their coding practices. Whether you are a student seeking coding assignment help or a professional working on enterprise applications, modular programming is a skill that will enhance your efficiency and productivity.
For personalized assistance with modular coding and best practices, explore online Programming Assignment Help services to gain expert guidance and ensure high-quality coding assignments.
Frequently Asked Questions (FAQs)
What is modular programming?
Modular programming is a software development technique where a program is divided into independent, reusable modules that handle specific tasks.
Why is modular code important?
Modular code improves readability, reusability, maintainability, and scalability, making it easier to develop and debug applications.
What are some best practices for writing modular code?
Best practices include following the Single Responsibility Principle, using encapsulation, maintaining a modular file structure, writing unit tests, and leveraging version control systems.
How does modular programming help in team collaboration?
Modular programming allows different developers to work on separate modules simultaneously, reducing dependencies and improving project efficiency.
What programming languages support modular programming?
Most modern programming languages, including Python, Java, C++, JavaScript, and C#, support modular programming principles.
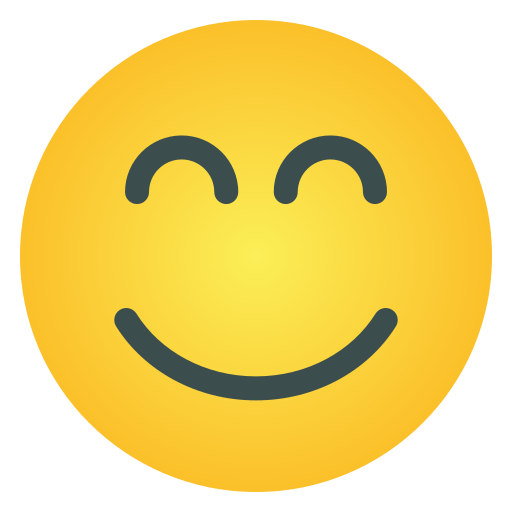